<java />
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
title: 'study',
color: Colors.grey,
home: loginPage(),
);
}
}
class loginPage extends StatefulWidget {
const loginPage({Key? key}) : super(key: key);
@override
State<loginPage> createState() => _loginPageState();
}
class _loginPageState extends State<loginPage> {
TextEditingController controller = TextEditingController();
TextEditingController controller2 = TextEditingController();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text("Log in Page"),
centerTitle: true,
leading: IconButton(
icon: Icon(Icons.menu),
onPressed: () {
;
},
),
actions: [
IconButton(
onPressed: () {
;
},
icon: Icon(Icons.search))
],
),
body: Builder(builder: (context) {
return SingleChildScrollView(
child: Column(
children: [
Padding(padding: EdgeInsets.only(top: 20)),
Center(
child: Image(
image: AssetImage('images/human1.png'),
width: 200,
),
),
Form(
child: Theme(
data: ThemeData(
primaryColor: Colors.teal,
inputDecorationTheme: InputDecorationTheme(
labelStyle: TextStyle(color: Colors.teal))),
child: Container(
padding: EdgeInsets.all(40),
child: Column(
children: [
TextField(
decoration: InputDecoration(labelText: "ID"),
keyboardType: TextInputType.emailAddress,
controller: controller,
),
TextField(
decoration:
InputDecoration(labelText: "PassWord"),
keyboardType: TextInputType.text,
obscureText: true,
controller: controller2,
),
SizedBox(
height: 40,
),
ButtonTheme(
minWidth: 120,
height: 60,
child: RaisedButton(
onPressed: () {
if (controller.text == 'tstory' &&
controller2.text == '1234') {
Navigator.push(
context,
MaterialPageRoute(
builder: (context) =>
HellowWorldPage()));
} else {
ShowSnakbar(context);
}
},
child: Icon(
Icons.arrow_forward_rounded,
size: 35,
color: Colors.white,
),
),
)
],
),
)))
],
),
);
}),
);
}
}
void ShowSnakbar(BuildContext context) {
Scaffold.of(context).showSnackBar(SnackBar(
content: Text(
"wrong",
style: TextStyle(
color: Colors.yellow, fontWeight: FontWeight.bold, fontSize: 20),
textAlign: TextAlign.center,
),
duration: Duration(seconds: 10),
backgroundColor: Colors.black,
));
}
class HellowWorldPage extends StatelessWidget {
const HellowWorldPage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Hellow World'),
centerTitle: true,
),
);
}
}
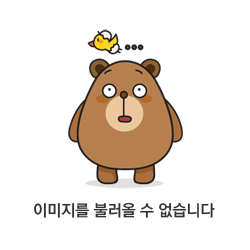